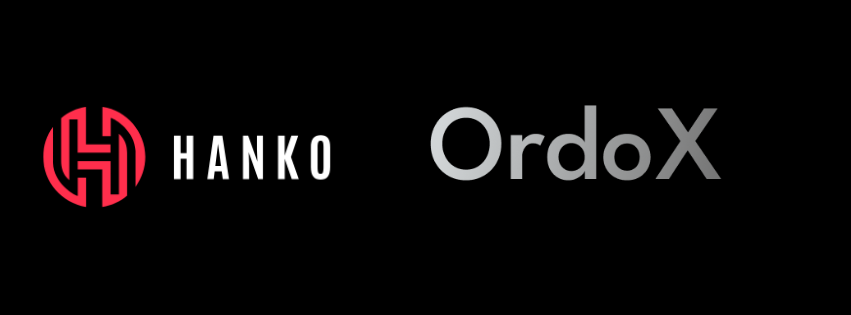
In the last blog, we talked about OrdoX and how we created an Unstructured data to JSON application. If you haven't read that yet you can read it here.
Although, we had a rate limited route we still didn't have any sort of Authentication which was necessary if we wanted to display our users with the audit logs for their requests.
Since passkeys are good for both user convenience and security I decided to search for Passkey based authentication services.
That's when I discovered Hanko. So it basically lets you create and passkey authentication with pretty much drag and drop components.
Let's quickly go through how we built it.
1. Create Hanko Account:
This is probably the only thing you need to setup. After you have successfully created an account grab the API URL
from the dashboard.
Let's add it in the env:
NEXT_PUBLIC_HANKO_API_URL=
Also, you will need to add the URL for your website. For development add http://localhost:3000/
2. Install Hanko and Use Elements:
Since our app is API first application we decided to go with Hanko Elements as it is the fastest way to build the authentication.
It’s pretty much plug and play. Let's install it:
pnpm add @teamhanko/hanko-elements
Once you have it installed you can easily add the components in your components directory. Refer to the Hanko docs for the components.
After that just add the component in your login/page.tsx or similar file:
'use client'
import HankoAuth from "@/components/HankoAuth";
import { useRouter } from 'next/navigation';
export default function LoginPage() {
const router = useRouter();
return (
<div className="flex flex-col items-center justify-center min-h-screen">
<div
className="absolute top-4 left-4 px-4 py-2 cursor-pointer"
onClick={()=> router.push('/')}
>
Back
</div>
<HankoAuth />
</div>
);
}
Here is the BEST part about Hanko. Unlike other authentication providers, Hanko allows you to customize these components. I wanted it to have black and white theme so I just changed their default variables to match that theme.
These are all the variables you can change to customize the styles:
hanko-auth,
hanko-profile {
/* Color Scheme */
--color: #333333;
--color-shade-1: #8f9095;
--color-shade-2: #e5e6ef;
--brand-color: black;
--brand-color-shade-1: black;
--brand-contrast-color: white;
--background-color: white;
--error-color: #e82020;
--link-color: black;
/* Font Styles */
--font-weight: 400;
--font-size: 16px;
--font-family: sans-serif;
/* Border Styles */
--border-radius: 50px;
--border-style: solid;
--border-width: 1px;
/* Item Styles */
--item-height: 34px;
--item-margin: 0.5rem 0;
/* Container Styles */
--container-padding: 30px;
--container-max-width: 410px;
/* Headline Styles */
--headline1-font-size: 24px;
--headline1-font-weight: 600;
--headline1-margin: 0 0 1rem;
--headline2-font-size: 16px;
--headline2-font-weight: 600;
--headline2-margin: 1rem 0 0.5rem;
/* Divider Styles */
--divider-padding: 0 42px;
--divider-visibility: visible;
/* Link Styles */
--link-text-decoration: none;
--link-text-decoration-hover: underline;
/* Input Styles */
--input-min-width: 14em;
/* Button Styles */
--button-min-width: max-content;
}
The above styling got me this customized card.
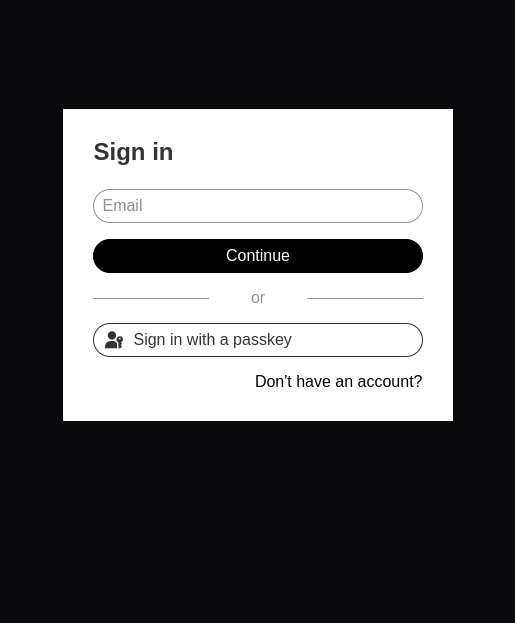
You can similarly create and use Logout buttons and User profiles.
3. Add middleware:
In the root directory just add the middleware given by Hanko. Don't forget to change the matcher
to actually match the routes of your application.
export const config = {
matcher: ["/dashboard"],
};
4. Get user data:
My application requires userId as we associate audit logs with it. This is how I did with Hanko. Add the hook from the docs and start using it.
import { useUserData } from "@/hooks/useUserdata";
const { id } = useUserData();
That's it. It was that simple to get the user data.
Wrapping Up:
In under an hour or so, I was able to build and deploy the entire authentication for this application. Without Hanko it would have take hours to build an authentication of this quality and security. Don't forget to check out Hanko and my app OrdoX